Facebook Hacker Cup Basketball Game Solution
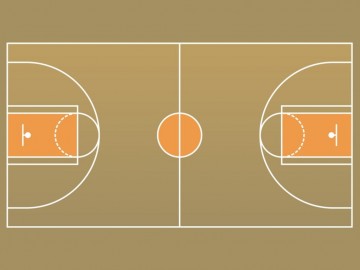
A group of N high school students wants to play a basketball game. To divide themselves into two teams they first rank all the players in the following way:
- Players with a higher shot percentage are rated higher than players with a lower shot percentage.
- If two players have the same shot percentage, the taller player is rated higher.
Luckily there are no two players with both the same shot percentage and height so they are able to order themselves in an unambiguous way. Based on that ordering each player is assigned a draft number from the range [1..N], where the highest-rated player gets the number 1, the second highest-rated gets the number 2, and so on. Now the first team contains all the players with the odd draft numbers and the second team all the players with the even draft numbers.
Each team can only have P players playing at a time, so to ensure that everyone gets similar time on the court both teams will rotate their players according to the following algorithm:
- Each team starts the game with the P players who have the lowest draft numbers.
- If there are more than P players on a team after each minute of the game the player with the highest total time played leaves the playing field. Ties are broken by the player with the higher draft number leaving first.
- To replace her the player on the bench with the lowest total time played joins the game. Ties are broken by the player with the lower draft number entering first.
The game has been going on for M minutes now. Your task is to print out the names of all the players currently on the field, (that is after M rotations).
Input
The first line of the input consists of a single number T, the number of test cases.
Each test case starts with a line containing three space separated integers N M P
The subsequent N lines are in the format “<name> <shot_percentage> <height>”. See the example for clarification.
Constraints
1 ≤ T ≤ 50
2 * P ≤ N ≤ 30
1 ≤ M ≤ 120
1 ≤ P ≤ 5
Each name starts with an uppercase English letter, followed by 0 to 20 lowercase English letters. There can be players sharing the same name. Each shot percentage is an integer from the range [0..100]. Each height is an integer from the range [100..240]
Output
For each test case i numbered from 1 to T, output “Case #i: “, followed by 2 * P space separated names of the players playing after M rotations. The names should be printed in lexicographical order.
Solution
<?php
class Player {
var $name;
var $shot_pct,$heigth,$time;
var $in;
function __construct($name, $shot_pct, $height){
$this->name=$name;
$this->shot_pct=$shot_pct;
$this->height=$height;
$this->in=FALSE;
$this->time=0;
}
}
function compare($a, $b)
{
if($a->shot_pct != $b->shot_pct) {
return $b->shot_pct - $a->shot_pct;
}
return $b->heigth - $a->heigth;
}
function compareNames($a, $b)
{
return strcmp($a->name, $b->name);
}
function getMin($p, $n)
{
$min = -1;
for($i = 0; $i < $n; $i++){
if($p[$i]->in == false && ($min == -1 || $p[$i]->time < $p[$min]->time)){
$min = $i;
}
}
return $min;
}
function getMax($p, $n)
{
$max = -1;
for($i = $n-1; $i >= 0; $i--){
if($p[$i]->in == true && ($max == -1 || $p[$i]->time > $p[$max]->time)){
$max = $i;
}
}
return $max;
}
$output='';
$cont = 0;
// get and import the input file
if(isset($_GET['file'])) { $file = $_GET['file']; } else { $file="input.txt"; }
$input = file("C:\\Users\\NEO\\Downloads\\".$file);
//$input = file("input.txt");
$test_cases = reset($input);
$output = '';
//Skip key 0 as it is the number of test cases!, start looping from 1
for ($case_number = 1, $t=1; $t<count($input); $t++) {
$n=$m=$p=0;
unset($i); unset($n1); unset($n2); unset($maxTime1);
unset($minTime1); unset($maxTime2); unset($minTime2);
unset($team1); unset($team2); $players_arr=array();
unset($player_data);
//echo "t:{$t}<br />";
//get numbers
$numbers = $input[$t];
list($n, $m, $p) = sscanf($numbers, "%d %d %d\n");
//slice out the player lines without preserving keys
$player_data = array_slice($input, $t+1, $n, FALSE);
for($i = 0; $i < $n; $i++)
{
list($name, $pct, $height) = sscanf($player_data[$i], "%s %d %d");
$player = new Player($name, $pct, $height);
$players_arr[]=$player;
}
usort($players_arr, "compare");
for($i = 0; $i < $n; $i+=2)
{
$team1[$i/2] = $players_arr[$i];
if($i+1 < $n) {
$team2[$i/2] = $players_arr[$i+1];
}
}
$n1 = intval(($n+1)/2);
$n2 = intval($n/2);
for($i = 0; $i < $p; $i++){
$team1[$i]->in = $team2[$i]->in = true;
}
while($m--)
{
for($i = 0; $i < $n1; $i++) {
if($team1[$i]->in){
$team1[$i]->time++;
}
}
for($i = 0; $i < $n2; $i++){
if($team2[$i]->in){
$team2[$i]->time++;
}
}
$minTime1 = getMin($team1, $n1);
$maxTime1 = getMax($team1, $n1);
if($minTime1 != -1)
{
$team1[$minTime1]->in = true;
$team1[$maxTime1]->in = false;
}
$minTime2 = getMin($team2, $n2);
$maxTime2 = getMax($team2, $n2);
if($minTime2 != -1)
{
$team2[$minTime2]->in = true;
$team2[$maxTime2]->in = false;
}
}
for($i = 0; $i < $n; $i += 2)
{
$players_arr[$i] = $team1[$i/2];
if($i+1 < $n)
$players_arr[$i+1] = $team2[$i/2];
}
usort($players_arr, "compareNames");
$output.="Case #".++$cont.":";
for($i = 0; $i < $n; $i++){
if($players_arr[$i]->in) {
$output.=" ".$players_arr[$i]->name;
}
}
if($test_cases<>$cont){
$output.=PHP_EOL;
}
$t=$t+$n;//+1;
}
// Save results to output file
file_put_contents('output.txt', $output);
?>
Example
In the first example if you sort all the players by their shot percentage you get the list: [Wai, Purav, Weiyan, Slawek, Lin, Meihong]. This makes the two teams:
[Wai, Weiyan, Lin]
[Purav, Slawek, Meihong]
The game starts with Lin and Meihong sitting on the bench in their respective teams. After the first minute passes it’s time for Weiyan and Slawek to sit out since they have the highest draft numbers of the people who played. After the second minute passes Lin and Meihong will keep playing since they only played one minute so far and it’s Wai and Purav who have to sit out.
Finally after the third minute Lin and Maihong go back to the bench and all the players currently playing again are:
Purav Slawek Wai Weiyan
5 6 3 2 Wai 99 131 Weiyan 81 155 Lin 80 100 Purav 86 198 Slawek 80 192 Meihong 44 109 7 93 2 Paul 82 189 Kittipat 62 126 Thomas 17 228 Fabien 57 233 Yifei 65 138 Liang 92 100 Victor 53 124 6 62 3 Meihong 33 192 Duc 62 162 Wai 70 148 Fabien 19 120 Bhuwan 48 176 Vlad 30 225 8 59 3 Anil 38 180 Song 7 187 David 65 159 Lin 45 121 Ranjeeth 39 183 Torbjorn 26 181 Clifton 57 158 Phil 3 183 4 72 1 Anh 2 187 Erling 69 226 Purav 0 199 Zejia 29 163
Case #1: Purav Slawek Wai Weiyan Case #2: Fabien Kittipat Liang Paul Case #3: Bhuwan Duc Fabien Meihong Vlad Wai Case #4: Anil Lin Phil Ranjeeth Song Torbjorn Case #5: Erling Zejia