Laravel Eloquent Model Parent/Child Relationship with itself
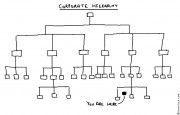
Unconditional love has a lot to do with a parents upbringing, or else you gotta tell them who their Daddy is! This relationship model helped me bring my SQL queries in my web page from over a 100 down to 6, it might help some people out:
note: the dependency_id column will contain the ID of the parent, you can modify this to have many to many relations using pivot tables!
<?php class Person extends \Eloquent { protected $fillable = []; var $mom, $kids; function __construct() { if($this->dependency_id<>0) { $this->mother->with('mother'); } } public function children() { $children = $this->hasMany('Person','dependency_id'); foreach($children as $child) { $child->mom = $this; } return $children; } public function mother() { $mother = $this->belongsTo('Person','dependency_id'); if(isset($mother->kids)) { $mother->kids->merge($mother); } return $mother; } public function canonical_url($type='soft') { if($type=='hard') { return Config::get('app.url'). (isset($this->mother->mother->slug)?$this->mother->mother->slug.'/':''). (isset($this->mother->slug)?$this->mother->slug.'/':''). $this->slug; }else { return Config::get('app.url'). (isset($this->mom->mom->slug)?$this->mom->mom->slug.'/':''). (isset($this->mom->slug)?$this->mom->slug.'/':''). $this->slug; } } }
Now you can do things like this:
<!--?php $page = Page::with('children','children.children')--->where('slug','=', $page_slug)->first(); ?> Then in your view file: <code><ul class="nav navbar-nav"> @foreach($navigation as $parent_item) <li class="@if(count($parent_item->children)) dropdown @endif @if($parent_item->canonical_url()==Request::path()) active @endif" > <a href="/{{$parent_item->slug}}"> {{$parent_item->name}} </a> @if(count($parent_item->children)) <ul class="dropdown-menu" role="menu" aria-labelledby="dLabel"> @foreach($parent_item->children as $child_item) <li > <a href="{{$child_item->canonical_url()}}"> {{$child_item->name}} <br> <span>{{$child_item->description}}</span> </a> @if(count($child_item->children)) <ul class="dropdown-menu"> <li data-bird='{{$child_item->summary}}'> <a href="{{$child_item->canonical_url()}}"> {{$child_item->title}} Overview </a> </li> @foreach($child_item->children as $grandchild_item) <li > <a href="{{$grandchild_item->canonical_url()}}"> {{$grandchild_item->name}} <br> <span>{{$grandchild_item->description}}</span> </a> </li> @endforeach </ul> @endif </li> @endforeach </ul> @endif </li> @endforeach </ul></code>